JavaScript: Get the sum of a given array using the provided function
JavaScript fundamental (ES6 Syntax): Exercise-32 with Solution
Sum Array After Mapping Function
Write a JavaScript program to get the sum of a given array, after mapping each element to a value using the provided function.
- Use Array.prototype.map() to map each element to the value returned by fn.
- Use Array.prototype.reduce() to add each value to an accumulator, initialized with a value of 0.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function named sumBy that calculates the sum of the values returned by applying a function to each element in an array.
const sumBy = (arr, fn) =>
// Map the array to extract the values based on the provided function or key.
arr.map(typeof fn === 'function' ? fn : val => val[fn])
// Reduce the mapped values to calculate their sum.
.reduce((acc, val) => acc + val, 0);
// Test the function with different arrays and functions/keys.
console.log(sumBy([{ n: 4 }, { n: 2 }, { n: 8 }, { n: 6 }], o => o.n)); // Output: 20
console.log(sumBy([{ n: -4 }, { n: -2 }, { n: 8 }, { n: 6 }], 'n')); // Output: 8
Output:
20 8
Visual Presentation:
Flowchart:
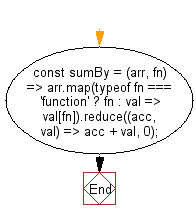
Live Demo:
See the Pen javascript-basic-exercise-1-32 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that maps each element of an array to a value using a provided function and returns their sum.
- Write a JavaScript function that applies a transformation to each array element before calculating the total using reduce().
- Write a JavaScript program that validates the mapping function’s output and then sums the resulting values.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to find all elements in an given array except for the first one. Return the whole array if the array's length is 1.
Next: Write a JavaScript program to get a random number in the specified range.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.