JavaScript: Filter out the element(s) of a given array, that have one of the specified values
JavaScript fundamental (ES6 Syntax): Exercise-30 with Solution
Filter Elements Matching Values
Write a JavaScript program to filter out the element(s) of a given array that have one of the specified values.
- Use Array.prototype.includes() to find values to exclude.
- Use Array.prototype.filter() to create an array excluding them.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function named without that filters out specified values from an array.
const without = (arr, ...args) => arr.filter(v => !args.includes(v));
// Test the function with different arrays and values to be filtered out.
console.log(without([2, 1, 2, 3], 1, 2)); // Output: [3]
console.log(without([2, 1, 2, 3], 3)); // Output: [2, 1, 2]
Output:
[3] [2,1,2]
Visual Presentation:
Flowchart:
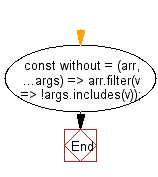
Live Demo:
See the Pen javascript-basic-exercise-1-30 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that removes all elements from an array that match any of the specified values.
- Write a JavaScript function that filters an array by excluding elements that exist in a given list of values.
- Write a JavaScript program that uses array filtering to return a new array without any of the unwanted specified values.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to convert a value to a safe integer.
Next: Write a JavaScript program to find all elements in an given array except for the first one. Return the whole array if the array's length is 1.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.