JavaScript: Find every element that exists in any of the two given arrays once
JavaScript fundamental (ES6 Syntax): Exercise-27 with Solution
Find Common Elements with Comparator
Write a JavaScript program to find every element in any of the two given arrays at once, using the provided comparator function.
- Create a new Set() with all values of a and values in b for which the comparator finds no matches in a, using Array.prototype.findIndex().
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `union_With` that computes the union of two arrays with a custom comparison function.
// It removes duplicates based on the comparison function provided.
const union_With = (a, b, comp) =>
Array.from(
new Set([
...a,
...b.filter(x => a.findIndex(y => comp(x, y)) === -1)
]));
// Example usage
console.log(union_With([1, 1.2, 1.5, 3, 0], [1.9, 3, 0, 3.9], (a, b) => Math.round(a) === Math.round(b)));
console.log(union_With([1, 2, 3, 4], [1, 2, 3, 4, 5], (a, b) => Math.round(a) === Math.round(b)));
Output:
[1,1.2,1.5,3,0,3.9] [1,2,3,4,5]
Flowchart:
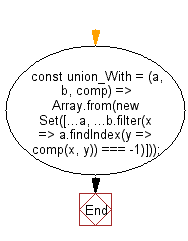
Live Demo:
See the Pen javascript-basic-exercise-1-27 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that finds common elements between two arrays using a custom comparator function.
- Write a JavaScript function that returns the intersection of two arrays by comparing elements with a provided comparator.
- Write a JavaScript program that iterates over two arrays and collects elements that satisfy the comparator condition for equality.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will return true if the string is y/yes or false if the string is n/no.
Next: Write a JavaScript program to measure the time taken by a function to execute.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics