JavaScript: Clamp a number within the inclusive range specified by the given boundary values a and b
JavaScript fundamental (ES6 Syntax): Exercise-266 with Solution
Clamp Number in Range
Write a JavaScript program to clamp a number within the inclusive range specified by the given boundary values a and b.
- If num falls within the range, return num.
- Otherwise, return the nearest number in the range.
Sample Solution:
JavaScript Code:
// Define a function 'clampNumber' to restrict a number within a specified range
const clampNumber = (num, a, b) =>
// Use Math.max and Math.min to ensure 'num' is within the range defined by 'a' and 'b'
Math.max(Math.min(num, Math.max(a, b)), Math.min(a, b));
// Test the function with different number and range combinations
console.log(clampNumber(2, 3, 5)); // Output: 3 (2 is clamped to the lower bound of the range)
console.log(clampNumber(1, -1, -5)); // Output: -1 (1 is clamped to the upper bound of the range)
Output:
3 -1
Flowchart:
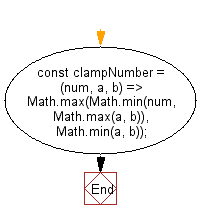
Live Demo:
See the Pen javascript-basic-exercise-266-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that clamps a number within a specified inclusive range using Math.min() and Math.max().
- Write a JavaScript function that takes a number and two boundary values, returning the number if within range or the nearest boundary otherwise.
- Write a JavaScript program that validates input and clamps a value to the lower or upper limit if it falls outside the range.
- Write a JavaScript function that demonstrates clamping by adjusting a series of numbers to lie within a given interval.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to chunk an array into smaller arrays of a specified size.
Next: Calculate the midpoint between two points.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.