JavaScript: Capitalize the first letter of a string
JavaScript fundamental (ES6 Syntax): Exercise-263 with Solution
Capitalize First Letter
Write a JavaScript program to capitalize the first letter of a string.
- Use array destructuring and String.prototype.toUpperCase() to capitalize the first letter of the string.
- Use Array.prototype.join('') to combine the capitalized first with the ...rest of the characters.
- Omit the lowerRest argument to keep the rest of the string intact, or set it to true to convert to lowercase.
Sample Solution:
JavaScript Code:
// Define a function 'capitalize' to capitalize the first letter of a string
// and optionally lowercase the rest of the string
const capitalize = ([first, ...rest], lowerRest = false) =>
// Convert the first character to uppercase and concatenate it with the rest of the string
first.toUpperCase() +
// If 'lowerRest' is true, join the remaining characters and convert them to lowercase,
// otherwise, join the remaining characters as they are
(lowerRest ? rest.join('').toLowerCase() : rest.join(''));
// Test the function with different inputs
console.log(capitalize('fooBar')); // Output: "FooBar"
console.log(capitalize('fooBar', true)); // Output: "Foobar"
Output:
FooBar Foobar
Visual Presentation:
Flowchart:
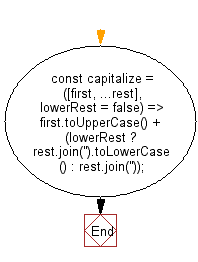
Live Demo:
See the Pen javascript-basic-exercise-263-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create a base-64 encoded ASCII string from a String object in which each character in the string is treated as a byte of binary data.
Next: Write a JavaScript program to capitalize the first letter of every word in a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics