JavaScript: Evaluate the binomial coefficient of two integers n and k
JavaScript fundamental (ES6 Syntax): Exercise-260 with Solution
Binomial Coefficient
Write a JavaScript program to evaluate the binomial coefficients of two integers n and k.
- Use Number.isNaN() to check if any of the two values is NaN.
- Check if k is less than 0, greater than or equal to n, equal to 1 or n - 1 and return the appropriate result.
- Check if n - k is less than k and switch their values accordingly.
- Loop from 2 through k and calculate the binomial coefficient.
- Use Math.round() to account for rounding errors in the calculation.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'binomialCoefficient' to calculate the binomial coefficient of two numbers 'n' and 'k'
const binomialCoefficient = (n, k) => {
// Return NaN if 'n' or 'k' is not a number
if (Number.isNaN(n) || Number.isNaN(k)) return NaN;
// Return 0 if 'k' is less than 0 or 'k' is greater than 'n'
if (k < 0 || k > n) return 0;
// Return 1 if 'k' is equal to 0 or 'k' is equal to 'n'
if (k === 0 || k === n) return 1;
// Return 'n' if 'k' is equal to 1 or 'k' is equal to 'n' - 1
if (k === 1 || k === n - 1) return n;
// If 'n - k' is less than 'k', assign 'n - k' to 'k'
if (n - k < k) k = n - k;
// Initialize 'res' to 'n'
let res = n;
// Loop from 2 to 'k' (inclusive)
for (let j = 2; j <= k; j++) {
// Calculate the binomial coefficient
res *= (n - j + 1) / j;
}
// Round the result and return
return Math.round(res);
};
// Calculate the binomial coefficient of 8 and 2
console.log(binomialCoefficient(8, 2)); // 28
Output:
28
Flowchart:
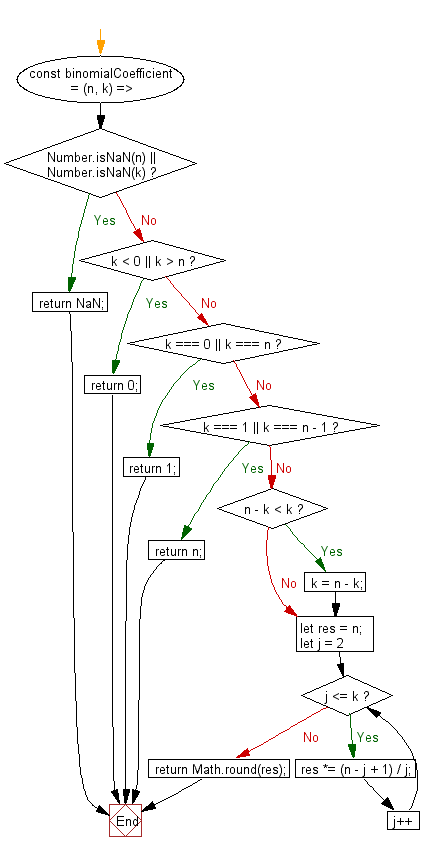
Live Demo:
See the Pen javascript-basic-exercise-260-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that calculates the binomial coefficient (n choose k) using recursion or dynamic programming.
- Write a JavaScript function that computes the binomial coefficient iteratively and handles large numbers gracefully.
- Write a JavaScript program that compares recursive and iterative implementations of n choose k and outputs their results.
- Write a JavaScript function that uses memoization to efficiently compute binomial coefficients for multiple inputs.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to decode a string of data which has been encoded using base-64 encoding.
Next: Write a JavaScript program that will return true if the bottom of the page is visible, false otherwise.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.