JavaScript: Create a function that accepts up to n arguments, ignoring any additional arguments
JavaScript fundamental (ES6 Syntax): Exercise-258 with Solution
Limit Function Arguments
Write a JavaScript program to create a function that accepts up to n arguments, ignoring any additional arguments.
- Call the provided function, fn, with up to n arguments, using Array.prototype.slice(0, n) and the spread operator (...).
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'ary' that takes a function 'fn' and a number 'n', returning a new function that accepts up to 'n' arguments
const ary = (fn, n) => (...args) => fn(...args.slice(0, n));
// Define a function 'firstTwoMax' that limits the maximum number of arguments to 2 using 'ary' with 'Math.max'
const firstTwoMax = ary(Math.max, 2);
// Map over an array of arrays, applying 'firstTwoMax' to each inner array
console.log([[2, 6, 'a'], [8, 4, 6], [10]].map(x => firstTwoMax(...x))); // [6, 8, 10] (maximum of the first two elements in each inner array)
Output:
[6,8,10]
Flowchart:
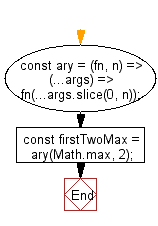
Live Demo:
See the Pen javascript-basic-exercise-257-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates a function wrapper limiting the number of arguments passed to the original function.
- Write a JavaScript function that accepts up to n arguments and ignores any extras using rest parameters.
- Write a JavaScript program that truncates the arguments list of a function call to a specified maximum number.
- Write a JavaScript function that returns a new function which only passes the first n arguments to the target function.
Go to:
PREV : 2D Array to CSV.
NEXT : Decode Base-64 String.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.