JavaScript: Return true if the provided predicate function returns true for at least one element in a collection, false otherwise
JavaScript fundamental (ES6 Syntax): Exercise-255 with Solution
Predicate True for Any
Write a JavaScript program that returns true if the provided predicate function returns true for at least one element in a collection, false otherwise.
- Use Array.prototype.some() to test if any elements in the collection return true based on fn.
- Omit the second argument, fn, to use Boolean as a default.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'any' that checks if any element in the array satisfies the provided condition
const any = (arr, fn = Boolean) => arr.some(fn);
// Test the 'any' function with different arrays and conditions
console.log(any([0, 1, 2, 0], x => x >= 2)); // true (at least one element is greater than or equal to 2)
console.log(any([0, 0, 1, 0])); // true (at least one element is truthy)
Output:
true true
Flowchart:
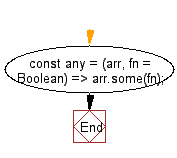
Live Demo:
See the Pen javascript-basic-exercise-255-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to generate a UUID in Node.JS. Use crypto API to generate a UUID, compliant with RFC4122 version 4.
Next: Write a JavaScript program to check if two given numbers are approximately equal to each other.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics