JavaScript: Write a JSON object to a file
JavaScript fundamental (ES6 Syntax): Exercise-251 with Solution
Write JSON to File
Write a JavaScript program to write a JSON object to a file.
- Use fs.writeFileSync(), template literals and JSON.stringify() to write a json object to a .json file.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Import the 'fs' module to work with the file system
const fs = require('fs');
// Define a function 'JSONToFile' that writes a JSON object to a file
const JSONToFile = (obj, filename) =>
// Write the JSON object to a file with the provided filename and '.json' extension
fs.writeFile(`${filename}.json`, JSON.stringify(obj, null, 2));
// Call 'JSONToFile' with a sample JSON object and filename
JSONToFile({ test: 'is passed' }, 'testJsonFile'); // writes the object to 'testJsonFile.json'
Flowchart:
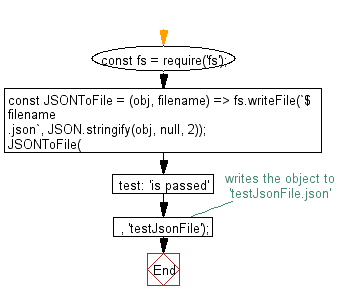
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create an element from a string (without appending it to the document).
Next: Write a JavaScript program to convert the values of RGB components to a color code.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics