JavaScript: Create an element from a string
JavaScript fundamental (ES6 Syntax): Exercise-250 with Solution
Create Element from String
Write a JavaScript program to create an element from a string (without appending it to the document).
If the given string contains multiple elements, only the first one will be returned.
- Use Document.createElement() to create a new element.
- Use Element.innerHTML to set its inner HTML to the string supplied as the argument.
- Use ParentNode.firstElementChild to return the element version of the string.
Sample Solution:
JavaScript Code:
Output:
container
Flowchart:
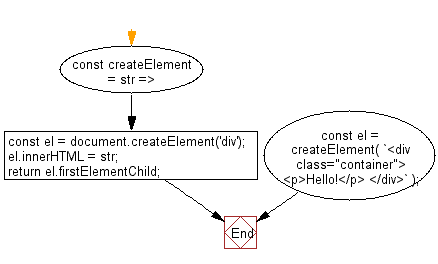
Live Demo:
For more Practice: Solve these Related Problems:
- Write a JavaScript program that creates a DOM element from an HTML string without appending it to the document.
- Write a JavaScript function that parses a string of HTML and returns the first element node found.
- Write a JavaScript program that converts a string to a DocumentFragment and extracts its child nodes.
- Write a JavaScript function that safely creates an element from a string and sets its innerHTML, then returns the element.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the current URL.
Next: Write a JavaScript program to write a JSON object to a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.