JavaScript: Deep flatten an array
JavaScript fundamental (ES6 Syntax): Exercise-248 with Solution
Write a JavaScript program to deep flatten an array.
- Use recursion.
- Use Array.prototype.concat() with an empty array ([]) and the spread operator (...) to flatten an array.
- Recursively flatten each element that is an array.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'deepFlatten' to recursively flatten nested arrays
const deepFlatten = arr => [].concat(...arr.map(v => (Array.isArray(v) ? deepFlatten(v) : v)));
// Call the 'deepFlatten' function with a nested array and log the flattened result
console.log(deepFlatten([1, [2], [[3], 4], 5]));
Output:
[1,2,3,4,5]
Flowchart:
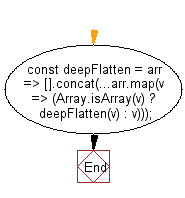
Live Demo:
See the Pen javascript-basic-exercise-248-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that Assigns default values for all properties in an object that are undefined.
Next: Write a JavaScript program to get the current URL.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-248.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics