JavaScript: Assigns default values for all properties in an object that are undefined
JavaScript fundamental (ES6 Syntax): Exercise-247 with Solution
Write a JavaScript program that assigns default values to all undefined properties in an object.
- Use Object.assign() to create a new empty object and copy the original one to maintain key order.
- Use Array.prototype.reverse() and the spread operator (...) to combine the default values from left to right.
- Finally, use obj again to overwrite properties that originally had a value.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'defaults' to merge multiple objects with the same keys, prioritizing the values from right to left
const defaults = (obj, ...defs) => Object.assign({}, obj, ...defs.reverse(), obj);
// Call the 'defaults' function with an object and multiple default objects, and log the merged result
console.log(defaults({ a: 1 }, { b: 2 }, { b: 6 }, { a: 3 }));
Output:
{"a":1,"b":2}
Flowchart:
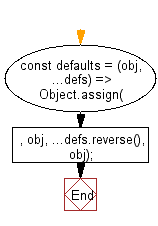
Live Demo:
See the Pen javascript-basic-exercise-247-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to convert an given angle from degrees to radians.
Next: Write a JavaScript program to deep flatten an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-247.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics