JavaScript: Invokes the provided function after wait milliseconds
JavaScript fundamental (ES6 Syntax): Exercise-245 with Solution
Invoke Function After Delay
Write a JavaScript program that invokes the provided function after a few milliseconds.
- Use setTimeout() to delay execution of fn.
- Use the spread (...) operator to supply the function with an arbitrary number of arguments.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'delay' to execute a function after a specified delay
const delay = (fn, wait, ...args) => setTimeout(fn, wait, ...args);
// Call the 'delay' function with a function to be executed, a delay of 1000ms, and additional arguments
delay(
function(text) {
console.log(text);
},
1000,
'later'
);
Output:
later
Flowchart:
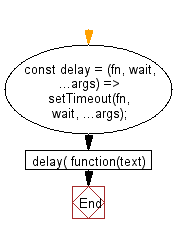
Live Demo:
See the Pen javascript-basic-exercise-245-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that invokes a given function after a specified number of milliseconds using setTimeout.
- Write a JavaScript function that delays the execution of a callback and logs the delay duration before calling it.
- Write a JavaScript program that schedules a function to run after a delay and returns a promise that resolves after execution.
- Write a JavaScript function that wraps a callback in a delayed execution context, allowing for asynchronous invocation.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the difference between two given arrays.
Next: Write a JavaScript program to convert an given angle from degrees to radians.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.