JavaScript: Get the difference between two given arrays
JavaScript fundamental (ES6 Syntax): Exercise-244 with Solution
Difference Between Arrays
Write a JavaScript program to get the difference between two given arrays.
- Create a Set from b to get the unique values in b.
- Use Array.prototype.filter() on a to only keep values not contained in b, using Set.prototype.has().
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'difference' to find the difference between two arrays
const difference = (a, b) => {
// Create a set 's' from array 'b'
const s = new Set(b);
// Return elements from array 'a' that are not present in set 's'
return a.filter(x => !s.has(x));
};
// Test the 'difference' function with different arrays
console.log(difference([1, 2, 3], [1, 2, 4])); // Output: [3]
console.log(difference([1, 2, 4], [1, 2, 3])); // Output: [4]
Output:
[3] [4]
Visual Presentation:
Flowchart:
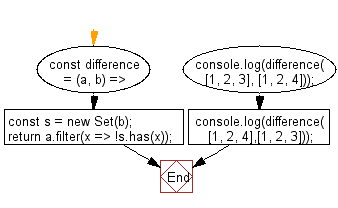
Live Demo:
See the Pen javascript-basic-exercise-244-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns an array of elements present in the first array but not in the second.
- Write a JavaScript function that computes the difference between two arrays using filtering and indexOf.
- Write a JavaScript program that compares two arrays and returns a new array containing their asymmetric difference.
- Write a JavaScript function that uses Set operations to determine the elements unique to each array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the distance between two given points.
Next: Write a JavaScript program to Invokes the provided function after wait milliseconds.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.