JavaScript: Return true if the parent element contains the child element, false otherwise
JavaScript fundamental (ES6 Syntax): Exercise-240 with Solution
Check Parent Contains Child
Write a JavaScript program that returns true if the parent element contains the child element, false otherwise.
Note: Check that parent is not the same element as child, use parent.contains(child) to check if the parent element contains the child element.
- Check that parent is not the same element as child.
- Use Node.contains() to check if the parent element contains the child element.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function 'elementContains' to check if a parent element contains a child element
const elementContains = (parent, child) =>
// Check if the parent element is not the same as the child element and if the parent contains the child
parent !== child && parent.contains(child);
// Test the 'elementContains' function with different parent-child element combinations
console.log(elementContains(document.querySelector('head'), document.querySelector('title'))); // Output: true
console.log(elementContains(document.querySelector('body'), document.querySelector('body'))); // Output: false
Output:
true false
Flowchart:
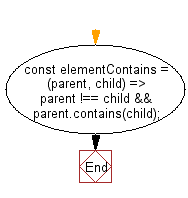
Live Demo:
See the Pen javascript-fundamental-exercise-240 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if a specified DOM element is contained within another element.
- Write a JavaScript function that uses Node.contains() to check for parent-child relationships in the DOM.
- Write a JavaScript program that traverses up the DOM tree to determine if an element is a descendant of a given parent.
- Write a JavaScript function that tests two DOM nodes and returns whether the first contains the second.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to escape a string to use in a regular expression.
Next: Write a JavaScript program to remove elements in an array until the passed function returns true. Returns the remaining elements in the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.