JavaScript: Decapitalize the first letter of a string
JavaScript fundamental (ES6 Syntax): Exercise-24 with Solution
Decapitalize First Letter of String
Write a JavaScript program to decapitalize the first letter of a string.
- Use array destructuring and String.prototype.toLowerCase() to decapitalize first letter, ...rest to get array of characters after first letter and then Array.prototype.join('') to make it a string again.
- Omit the upperRest argument to keep the rest of the string intact, or set it to true to convert to uppercase.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `decapitalize` that converts the first character of a string to lowercase and optionally converts the rest of the string to uppercase.
const decapitalize = ([first, ...rest], upperRest = false) =>
first.toLowerCase() + (upperRest ? rest.join('').toUpperCase() : rest.join(''));
// Example usage
console.log(decapitalize('W3resource'));
console.log(decapitalize('Red', true));
Note: Omit the upperRest parameter to keep the rest of the string intact, or set it to true to convert to uppercase
Output:
w3resource rED
Visual Presentation:
Flowchart:
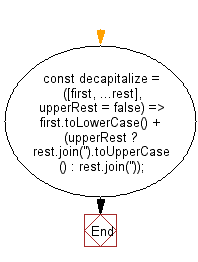
Live Demo:
See the Pen javascript-basic-exercise-1-24 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts the first character of a string to lowercase while leaving the rest unchanged.
- Write a JavaScript function that decapitalizes the initial letter of each word in a sentence.
- Write a JavaScript program that checks if the first character is uppercase and converts it to lowercase if needed.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to filter out the non-unique values in an array, based on a provided comparator function.
Next: Write a JavaScript program to create a new array out of the two supplied by creating each possible pair from the arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.