JavaScript: Get the first key that satisfies the provided testing function
JavaScript fundamental (ES6 Syntax): Exercise-236 with Solution
First Key Satisfying Condition
Write a JavaScript program to get the first key that satisfies the provided testing function. Otherwise return undefined.
- Use Object.keys(obj) to get all the properties of the object, Array.prototype.find() to test each key-value pair using fn.
- The callback receives three arguments - the value, the key and the object.
Sample Solution:
JavaScript Code:
// Define a function 'findKey' to find the first key in an object that satisfies a provided testing function
const findKey = (obj, fn) =>
Object.keys(obj).find(key => fn(obj[key], key, obj)); // Find the first key that satisfies the condition
// Test the 'findKey' function with a sample object and testing function
console.log(findKey(
{
barney: { age: 36, active: true },
fred: { age: 40, active: false },
pebbles: { age: 1, active: true }
},
o => o['active'] // Check if the 'active' property of the object is true
)); // Output: 'barney'
Output:
barney
Flowchart:
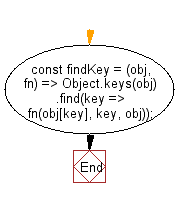
Live Demo:
See the Pen javascript-basic-exercise-236-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns the first key in an object that satisfies a given predicate function.
- Write a JavaScript function that iterates over an object's keys and returns the first key for which the callback is true.
- Write a JavaScript program that uses Object.keys() and Array.find() to retrieve the first matching key.
- Write a JavaScript function that searches through an object's properties and returns the key that first meets a condition.
Go to:
PREV : Last Key Satisfying Condition.
NEXT : Fibonacci Sequence.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.