JavaScript: Flatten a given array up to the specified depth
JavaScript fundamental (ES6 Syntax): Exercise-234 with Solution
Write a JavaScript program to flatten a given array to the specified depth.
- Use recursion, decrementing depth by 1 for each level of depth.
- Use Array.prototype.reduce() and Array.prototype.concat() to merge elements or arrays.
- Base case, for depth equal to 1 stops recursion.
- Write a JavaScript program to flatten a given array to the specified depth.
Sample Solution:
JavaScript Code:
// Define a function 'flatten' that flattens nested arrays up to a specified depth
const flatten = (arr, depth = 1) =>
arr.reduce((a, v) =>
a.concat(depth > 1 && Array.isArray(v) ? flatten(v, depth - 1) : v), []);
// Test the 'flatten' function with sample arrays
console.log(flatten([1, [2], 3, 4])); // Output: [1, 2, 3, 4]
console.log(flatten([1, [2, [3, [4, 5], 6], 7], 8], 2)); // Output: [1, 2, 3, [4, 5], 6, 7, 8]
Output:
[1,2,3,4] [1,2,3,[4,5],6,7,8]
Flowchart:
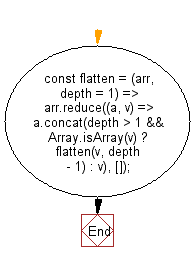
Live Demo:
See the Pen javascript-basic-exercise-234-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to flatten an object with the paths for keys.
Next: Write a JavaScript program to get the last key that satisfies the provided testing function, otherwise undefined is returned.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-234.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics