JavaScript: Flatten an object with the paths for keys
JavaScript fundamental (ES6 Syntax): Exercise-233 with Solution
Flatten Object Keys as Paths
Write a JavaScript program to flatten an object with the paths for keys.
- Use recursion.
- Use Object.keys(obj) combined with Array.prototype.reduce() to convert every leaf node to a flattened path node.
- If the value of a key is an object, the function calls itself with the appropriate prefix to create the path using Object.assign().
- Otherwise, it adds the appropriate prefixed key-value pair to the accumulator object. You should always omit the second argument, prefix, unless you want every key to have a prefix.
Sample Solution:
JavaScript Code:
// Define a function 'flattenObject' that flattens nested objects into a single-level object with dot-separated keys
const flattenObject = (obj, prefix = '') =>
Object.keys(obj).reduce((acc, k) => {
const pre = prefix.length ? prefix + '.' : ''; // Construct the prefix for the current key
if (typeof obj[k] === 'object') // If the current value is an object, recursively call 'flattenObject' and merge the result into the accumulator
Object.assign(acc, flattenObject(obj[k], pre + k));
else acc[pre + k] = obj[k]; // Otherwise, assign the current value to the accumulator with the constructed key
return acc; // Return the accumulator
}, {});
// Test the 'flattenObject' function with a sample object
console.log(flattenObject({ a: { b: { c: 1 } }, d: 1 }));
Output:
{"a.b.c":1,"d":1}
Flowchart:
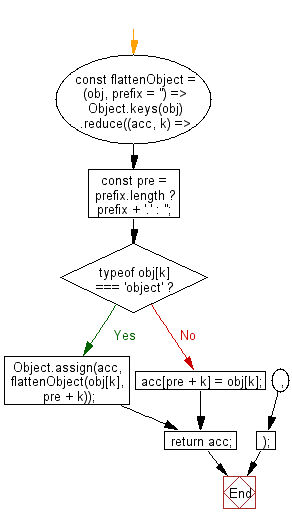
Live Demo:
See the Pen javascript-basic-exercise-233-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that flattens an object, converting nested keys into a single path string (e.g., "a.b.c").
- Write a JavaScript function that recursively traverses an object and produces a flat object with dotted key paths.
- Write a JavaScript program that converts a nested object into a single-level object with keys representing the full path.
- Write a JavaScript function that maps deep object properties to flat key strings using a delimiter of your choice.
Go to:
PREV : Move First Argument Last.
NEXT : Flatten Array to Depth.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.