JavaScript: Takes a function as an argument, then makes the first argument the last
JavaScript fundamental (ES6 Syntax): Exercise-232 with Solution
Move First Argument Last
Write a JavaScript program that takes a function as an argument, then makes the first argument the last.
- Use argument destructuring and a closure with variadic arguments.
- Splice the first argument, using the spread operator (...), to make it the last before applying the rest.
Sample Solution:
JavaScript Code:
// Define a function 'flip' that takes a function 'fn' as input and returns a new function that flips the first two arguments before calling 'fn'
const flip = fn => (first, ...rest) => fn(...rest, first);
// Initialize objects 'a' and 'b'
let a = { name: 'John Smith' };
let b = {};
// Define a function 'mergeFrom' using 'flip' and 'Object.assign' that merges the properties of the second object into the first object
const mergeFrom = flip(Object.assign);
// Bind 'mergeFrom' to 'a' to create a new function 'mergePerson' that merges properties from 'a' into another object
let mergePerson = mergeFrom.bind(null, a);
// Merge properties from 'a' into 'b' using the 'mergePerson' function
mergePerson(b); // == b
// Reinitialize object 'b'
b = {};
// Log the result of merging properties from 'a' into 'b' using 'Object.assign'
console.log(Object.assign(b, a)); // == b
Output:
{"name":"John Smith"}
Flowchart:
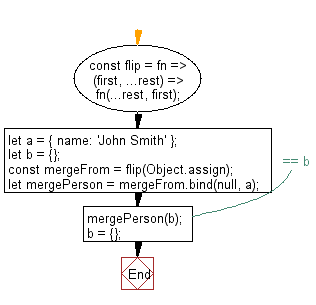
Live Demo:
See the Pen javascript-basic-exercise-232-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to iterate over all own properties of an object in reverse, running a callback for each one.
Next: Write a JavaScript program to flatten an object with the paths for keys.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics