JavaScript: Iterate over all own properties of an object in reverse, running a callback for each one
JavaScript fundamental (ES6 Syntax): Exercise-231 with Solution
Iterate Object Properties in Reverse
Write a JavaScript program to iterate over all the properties of an object in reverse, running a callback for each one.
- Use Object.keys(obj) to get all the properties of the object, Array.prototype.reverse() to reverse their order.
- Use Array.prototype.forEach() to run the provided function for each key-value pair.
- The callback receives three arguments - the value, the key and the object.
Sample Solution:
JavaScript Code:
// Define a function 'forOwnRight' that iterates over an object's own properties in reverse order and executes a function for each property
const forOwnRight = (obj, fn) =>
// Get the keys of the object, reverse their order, and iterate over them
Object.keys(obj)
.reverse()
.forEach(key => fn(obj[key], key, obj));
// Test the 'forOwnRight' function by logging the values of each property in reverse order
console.log(forOwnRight({ foo: 'bar', a: 1 }, v => console.log(v)));
Output:
1 bar undefined
Flowchart:
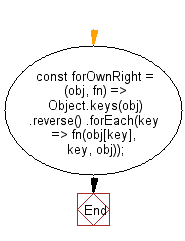
Live Demo:
See the Pen javascript-basic-exercise-231-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that iterates over an object's keys in reverse order and logs each key-value pair.
- Write a JavaScript function that retrieves an object's keys, reverses the order, and processes each property with a callback.
- Write a JavaScript program that uses Object.keys() and reverse() to iterate through properties backwards.
- Write a JavaScript function that returns an array of key-value pairs from an object in reverse key order.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the human readable format of the given number of milliseconds.
Next: Write a JavaScript program that takes a function as an argument, then makes the first argument the last.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics