JavaScript: Filter out the non-unique values in an array, based on a provided comparator function
JavaScript fundamental (ES6 Syntax): Exercise-23 with Solution
Filter Non-Unique with Comparator
Write a JavaScript program to filter out non-unique values in an array, based on a provided comparator function.
Note: The comparator function takes four arguments: the values of the two elements being compared and their indexes.
- Use Array.prototype.filter() and Array.prototype.every() to create an array containing only the unique values, based on the comparator function, fn.
- The comparator function takes four arguments: the values of the two elements being compared and their indexes.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `filter_Non_UniqueBy` that filters an array of objects based on a provided comparison function.
const filter_Non_UniqueBy = (arr, fn) =>
arr.filter((v, i) => arr.every((x, j) => (i === j) === fn(v, x, i, j)));
// Example usage
console.log(filter_Non_UniqueBy(
[
{ id: 0, value: 'a' },
{ id: 1, value: 'b' },
{ id: 2, value: 'c' },
{ id: 1, value: 'd' },
{ id: 0, value: 'e' }
],
(a, b) => a.id == b.id
)); // [{ id: 2, value: 'c' }]
Output:
[{"id":2,"value":"c"}]
Flowchart:
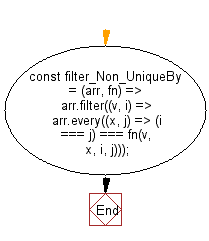
Live Demo:
See the Pen javascript-basic-exercise-1-23 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that filters out non-unique elements from an array based on a custom comparator function.
- Write a JavaScript function that compares array elements using a provided comparator to return only unique values.
- Write a JavaScript program that applies a comparator to determine and remove duplicate objects from an array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to filter out the non-unique values in an array.
Next: Write a JavaScript program to decapitalize the first letter of a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.