JavaScript: Convert a string from camelcase
JavaScript fundamental (ES6 Syntax): Exercise-229 with Solution
Camelcase to String
Write a JavaScript program to convert a string from camelcase.
- Use String.prototype.replace() to break the string into words and add a separator between them.
- Omit the second argument to use a default separator of _.
Sample Solution:
JavaScript Code:
// Define a function 'fromCamelCase' that converts a camelCase string to snake_case by inserting a separator between words
const fromCamelCase = (str, separator = '_') =>
// Replace occurrences where a lowercase character or digit is followed by an uppercase character with the same characters separated by the specified separator
// Replace occurrences where one or more consecutive uppercase characters are followed by an uppercase character followed by a lowercase character or digit with the same characters separated by the specified separator
// Convert the result to lowercase
str
.replace(/([a-z\d])([A-Z])/g, '$1' + separator + '$2')
.replace(/([A-Z]+)([A-Z][a-z\d]+)/g, '$1' + separator + '$2')
.toLowerCase();
// Test cases
console.log(fromCamelCase('someDatabaseFieldName', ' '));
console.log(fromCamelCase('someLabelThatNeedsToBeCamelized', '-'));
console.log(fromCamelCase('someJavascriptProperty', '_'));
Output:
some database field name some-label-that-needs-to-be-camelized some_javascript_property
Visual Presentation:
Flowchart:
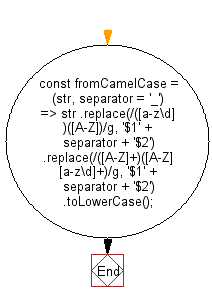
Live Demo:
See the Pen javascript-basic-exercise-229-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to log the name of a function.
Next: Write a JavaScript program to get the human readable format of the given number of milliseconds.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics