JavaScript: Get n random elements at unique keys from array up to the size of array
JavaScript fundamental (ES6 Syntax): Exercise-226 with Solution
n Random Unique Elements
Write a JavaScript program to get n random elements with unique keys from an array up to the size of the array.
- Shuffle the array using the Fisher-Yates algorithm.
- Use Array.prototype.slice() to get the first n elements.
- Omit the second argument, n, to get only one element at random from the array.
Sample Solution:
JavaScript Code:
// Define a function 'sampleSize' to randomly sample elements from an array
const sampleSize = ([...arr], n = 1) => {
let m = arr.length;
// Shuffle the array using the Fisher-Yates algorithm
while (m) {
const i = Math.floor(Math.random() * m--);
[arr[m], arr[i]] = [arr[i], arr[m]];
}
// Return a slice of the shuffled array with 'n' elements
return arr.slice(0, n);
};
// Log the result of sampling 2 elements from an array
console.log(sampleSize([1, 2, 3], 2));
// Log the result of sampling 4 elements from an array (exceeding the array length)
console.log(sampleSize([1, 2, 3], 4));
Output:
[3,2] [2,3,1]
Visual Presentation:
Flowchart:
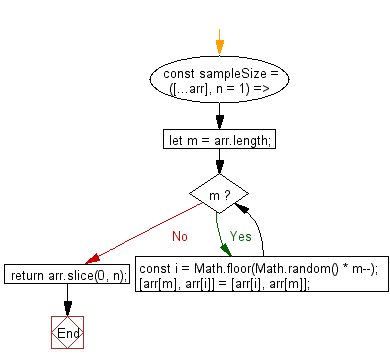
Live Demo:
See the Pen javascript-basic-exercise-226-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns n random elements from an array ensuring no duplicates are selected.
- Write a JavaScript function that shuffles an array and then extracts the first n elements for uniqueness.
- Write a JavaScript program that uses a Set to keep track of chosen elements until n unique items are gathered.
- Write a JavaScript function that validates n against the array length and returns an array of n unique random items.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get n random elements at unique keys from array up to the size of array.
Next: Write a JavaScript program to remove elements from an array for which the given function returns false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.