JavaScript: Initializes an array containing the numbers in the specified range
JavaScript fundamental (ES6 Syntax): Exercise-222 with Solution
Range Array with Ratio Step
Write a JavaScript program that initializes an array containing the numbers in the specified range. This is where start and end are inclusive and the ratio between the two terms is step. Returns an error if step equals 1.
- Use Array.from(), Math.log() and Math.floor() to create an array of the desired length, Array.prototype.map() to fill with the desired values in a range.
- Omit the second argument, start, to use a default value of 1.
- Omit the third argument, step, to use a default value of 2.
Sample Solution:
JavaScript Code:
// Define a function 'geometricProgression' that generates a geometric progression sequence
const geometricProgression = (end, start = 1, step = 2) =>
// Create an array based on the length of the geometric progression sequence
Array.from({ length: Math.floor(Math.log(end / start) / Math.log(step)) + 1 }).map(
// Generate each element of the sequence based on the start, step, and index
(v, i) => start * step ** i
);
// Log the geometric progression sequences with different parameters
console.log(geometricProgression(256));
console.log(geometricProgression(256, 3));
console.log(geometricProgression(256, 1, 4));
Output:
[1,2,4,8,16,32,64,128,256] [3,6,12,24,48,96,192] [1,4,16,64,256]
Pictorial Presentation:
Flowchart:
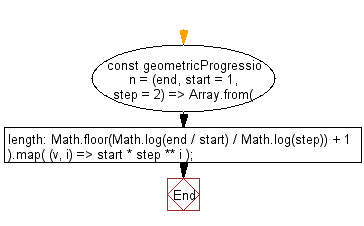
Live Demo:
See the Pen javascript-basic-exercise-222-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get a string of the form HH:MM:SS from a Date object.
Next: Write a JavaScript program to calculate the greatest common divisor between two or more numbers/arrays.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics