JavaScript: Get the native type of a value
JavaScript fundamental (ES6 Syntax): Exercise-220 with Solution
Get Native Type of Value
Write a JavaScript program to get the native type of a value. Returns the lowercased constructor name of value, "undefined" or "null" if value is undefined or null.
- Return 'undefined' or 'null' if the value is undefined or null.
- Otherwise, use Object.prototype.constructor.name to get the name of the constructor.
Sample Solution:
JavaScript Code:
// Define a function 'getType' to determine the type of a value
const getType = v =>
// Check if the value is undefined and return 'undefined' if true
v === undefined ? 'undefined' :
// Check if the value is null and return 'null' if true
v === null ? 'null' :
// If the value is not undefined or null, return the lowercase name of its constructor
v.constructor.name.toLowerCase();
// Test the 'getType' function with a Set object
console.log(getType(new Set([1, 2, 3]))); // Output: 'set'
Output:
set
Flowchart:
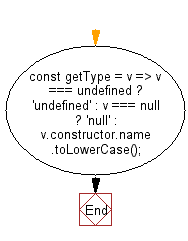
Live Demo:
See the Pen javascript-basic-exercise-220-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns the native type of a value by examining its constructor name in lowercase.
- Write a JavaScript function that uses Object.prototype.toString() to determine the precise type of a variable.
- Write a JavaScript program that distinguishes between primitive types and objects by returning their native type names.
- Write a JavaScript function that handles null and undefined values, returning "null" or "undefined" as appropriate.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to calculate the Hamming distance between two values.
Next: Write a JavaScript program to get a string of the form HH:MM:SS from a Date object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics