JavaScript: Calculate the Hamming distance between two values
JavaScript fundamental (ES6 Syntax): Exercise-219 with Solution
Hamming Distance
Write a JavaScript program to calculate the Hamming distance between two values.
- Use the XOR operator (^) to find the bit difference between the two numbers.
- Convert to a binary string using Number.prototype.toString(2).
- Count and return the number of 1s in the string, using String.prototype.match(/1/g).
Sample Solution:
JavaScript Code:
// Define a function 'hammingDistance' to calculate the Hamming distance between two numbers
const hammingDistance = (num1, num2) => (
// XOR the two numbers and convert the result to a binary string
(num1 ^ num2)
.toString(2)
// Match all occurrences of '1' in the binary string and return their count, which represents the Hamming distance
.match(/1/g) || ''
).length;
// Test the 'hammingDistance' function with different pairs of numbers
console.log(hammingDistance(2, 3)); // Output: 1
console.log(hammingDistance(5, 3)); // Output: 2
// Define a function 'hammingDistance' to calculate the Hamming distance between two numbers
const hammingDistance = (num1, num2) => (
// XOR the two numbers and convert the result to a binary string
(num1 ^ num2)
.toString(2)
// Match all occurrences of '1' in the binary string and return their count, which represents the Hamming distance
.match(/1/g) || ''
).length;
// Test the 'hammingDistance' function with different pairs of numbers
console.log(hammingDistance(2, 3)); // Output: 1
console.log(hammingDistance(5, 3)); // Output: 2
Output:
1 2
Flowchart:
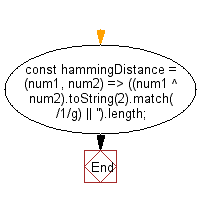
Live Demo:
See the Pen javascript-basic-exercise-219-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the number of times a function executed per second.
Next: Write a JavaScript program to get the native type of a value. Returns lowercased constructor name of value, "undefined" or "null" if value is undefined or null.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics