JavaScript: Initializes an array containing the numbers in the specified range (in reverse) where start and end are inclusive with their common difference step
JavaScript fundamental (ES6 Syntax): Exercise-214 with Solution
Write a JavaScript program to initialize an array containing the numbers in the specified range (in reverse) where start and end are inclusive of their common difference step.
- Use Array.from(Math.ceil((end+1-start)/step)) to create an array of the desired length(the amounts of elements is equal to (end-start)/step or (end+1-start)/step for inclusive end), Array.prototype.map() to fill with the desired values in a range.
- Omit the second argument, start, to use a default value of 0.
- Omit the last argument, step, to use a default value of 1.
Sample Solution:
JavaScript Code:
// Define a function 'initializeArrayWithRangeRight' that creates an array filled with a range of numbers, starting from 'start' (default: 0) to 'end' (inclusive), with a specified 'step' (default: 1), in reverse order
const initializeArrayWithRangeRight = (end, start = 0, step = 1) =>
// Create an array of length equal to the number of elements in the range using 'Array.from'
Array.from({ length: Math.ceil((end + 1 - start) / step) })
// Map each element in the array to the corresponding value in the range, starting from 'end' and decrementing by 'step'
.map((v, i, arr) => (arr.length - i - 1) * step + start);
// Test the 'initializeArrayWithRangeRight' function by creating arrays filled with ranges of numbers in reverse order
console.log(initializeArrayWithRangeRight(5));
// Output: [5, 4, 3, 2, 1, 0]
console.log(initializeArrayWithRangeRight(7, 3));
// Output: [7, 6, 5, 4, 3]
console.log(initializeArrayWithRangeRight(9, 0, 2));
// Output: [8, 6, 4, 2, 0]
Output:
[5,4,3,2,1,0] [7,6,5,4,3] [8,6,4,2,0]
Visual Presentation:
Flowchart:
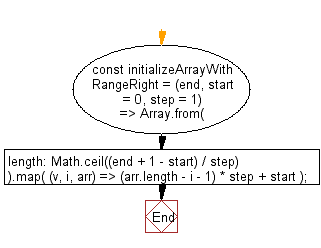
Live Demo:
See the Pen javascript-fundamental-exercise-214 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to initialize and fill an array with the specified values.
Next: Write a JavaScript program to get all the elements of an array except the last one.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-214.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics