JavaScript: Create a n-dimensional array with given value
JavaScript fundamental (ES6 Syntax): Exercise-212 with Solution
Write a JavaScript program to create a n-dimensional array with a given value.
- Use recursion.
- Use Array.from(), Array.prototype.map() to generate rows where each is a new array initialized using initializeNDArray().
Sample Solution:
JavaScript Code:
// Define a function 'initializeNDArray' that creates a multi-dimensional array with a specified value
const initializeNDArray = (val, ...args) =>
// If no dimensions are provided, return the specified value
args.length === 0
? val
// If dimensions are provided, recursively create multi-dimensional arrays
: Array.from({ length: args[0] }).map(() => initializeNDArray(val, ...args.slice(1)));
// Test the 'initializeNDArray' function by creating multi-dimensional arrays with specified values
console.log(initializeNDArray(1, 3));
// Output: [1, 1, 1]
console.log(initializeNDArray(5, 2, 2, 2));
// Output: [[[5, 5], [5, 5]], [[5, 5], [5, 5]]]
Output:
[1,1,1] [[[5,5],[5,5]],[[5,5],[5,5]]]
Visual Presentation:
Flowchart:
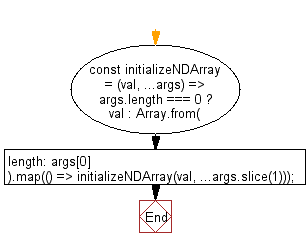
Live Demo:
See the Pen javascript-basic-exercise-212-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get a list of elements that exist in both arrays.
Next: Write a JavaScript program to initialize and fill an array with the specified values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/javascript-exercises/fundamental/javascript-fundamental-exercise-212.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics