JavaScript: Get every nth element in a given array
JavaScript fundamental (ES6 Syntax): Exercise-21 with Solution
Every nth Element in Array
Write a JavaScript program to get every nth element in a given array.
- Use Array.prototype.filter() to create a new array that contains every nth element of a given array.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
// Define a function called `every_nth` that filters an array and returns every nth element.
const every_nth = (arr, nth) => arr.filter((e, i) => i % nth === nth - 1);
// Example usage
console.log(every_nth([1, 2, 3, 4, 5, 6], 1)); // [1, 2, 3, 4, 5, 6]
console.log(every_nth([1, 2, 3, 4, 5, 6], 2)); // [2, 4, 6]
console.log(every_nth([1, 2, 3, 4, 5, 6], 3)); // [3, 6]
console.log(every_nth([1, 2, 3, 4, 5, 6], 4)); // [4]
Output:
[1,2,3,4,5,6] [2,4,6] [3,6] [4]
Visual Presentation:
Flowchart:
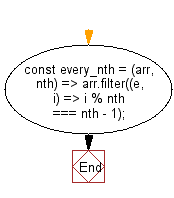
Live Demo:
See the Pen javascript-basic-exercise-1-21 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns every nth element from an array using a loop or functional approach.
- Write a JavaScript function that filters an array to include only elements at positions that are multiples of n.
- Write a JavaScript program that slices an array based on a given interval and returns the resulting elements.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to extend a 3-digit color code to a 6-digit color code.
Next: Write a JavaScript program to filter out the non-unique values in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.