JavaScript: Check whether the provided value is of the specified type
JavaScript fundamental (ES6 Syntax): Exercise-208 with Solution
Check Specified Type
Write a JavaScript program to check whether the provided value is of the specified type.
- Ensure the value is not undefined or null using Array.prototype.includes().
- Compare the constructor property on the value with type to check if the provided value is of the specified type.
Sample Solution:
JavaScript Code:
// Define a function 'is' that checks if the provided value is an instance of the specified type
const is = (type, val) => ![, null].includes(val) && val.constructor === type;
// Test cases to check if the provided values are instances of the specified types
console.log(is(Array, [1])); // true (Array instance)
console.log(is(ArrayBuffer, new ArrayBuffer())); // true (ArrayBuffer instance)
console.log(is(Map, new Map())); // true (Map instance)
console.log(is(RegExp, /./g)); // true (RegExp instance)
console.log(is(Set, new Set())); // true (Set instance)
console.log(is(WeakMap, new WeakMap())); // true (WeakMap instance)
console.log(is(WeakSet, new WeakSet())); // true (WeakSet instance)
console.log(is(String, '')); // true (String instance)
console.log(is(String, new String(''))); // true (String instance)
console.log(is(Number, 1)); // true (Number instance)
console.log(is(Number, new Number(1))); // true (Number instance)
console.log(is(Boolean, true)); // true (Boolean instance)
console.log(is(Boolean, new Boolean(true))); // true (Boolean instance)
Output:
true true true true true true true true true true true true true
Flowchart:
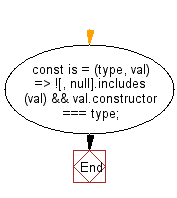
Live Demo:
See the Pen javascript-basic-exercise-208-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if a provided value is of a specified type using typeof and constructor comparisons.
- Write a JavaScript function that accepts a value and a type string, then returns true if the value matches that type.
- Write a JavaScript program that validates an input’s type against a given string such as "string", "number", or "object".
- Write a JavaScript function that uses Object.prototype.toString to check if a value is of the specified type.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to that will return true if the given string is an absolute URL, false otherwise.
Next: Write a JavaScript program to get a list of elements that exist in both arrays, using a provided comparator function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.