JavaScript: Return true if the given string is an absolute URL, false otherwise
JavaScript fundamental (ES6 Syntax): Exercise-207 with Solution
Check Absolute URL
Write a JavaScript program that returns true if the given string is an absolute URL, false otherwise.
- Use RegExp.prototype.test() to test if the string is an absolute URL.
Sample Solution:
JavaScript Code:
Output:
true true false
Flowchart:
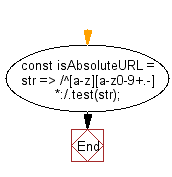
Live Demo:
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if a string starts with a valid protocol (http, https, ftp) indicating an absolute URL.
- Write a JavaScript function that uses regular expressions to test if a URL is absolute.
- Write a JavaScript program that checks if a URL string contains a valid domain and protocol.
- Write a JavaScript function that validates URL format and returns true if it’s an absolute URL.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to check if a given string is an anagram of another string (case-insensitive, ignores spaces, punctuation and special characters).
Next: Write a JavaScript program to check whether the provided value is of the specified type.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.