JavaScript: Check whether the provided argument is array-like
JavaScript fundamental (ES6 Syntax): Exercise-205 with Solution
Check If Array
Write a JavaScript program to check if the provided argument is an array (i.e. iterable).
- Check if the provided argument is not null and that its Symbol.iterator property is a function.
Sample Solution:
JavaScript Code:
// Define a function 'isArrayLike' that checks if the input value is array-like
const isArrayLike = val => {
try {
return [...val], true; // Try to spread the input value into an array and return true
} catch (e) {
return false; // If an error occurs, return false
}
};
// Test cases to check if the input values are array-like
console.log(isArrayLike(document.querySelectorAll('.className'))); // true (document.querySelectorAll('.className') returns a NodeList which is array-like)
console.log(isArrayLike('abc')); // true ('abc' is a string which is array-like)
console.log(isArrayLike(null)); // false (null is not array-like)
Output:
true true false
Flowchart:
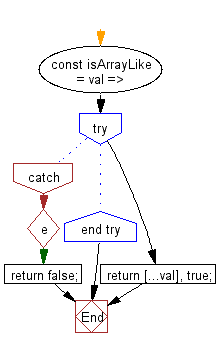
Live Demo:
See the Pen javascript-basic-exercise-205-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if the provided argument is an array using Array.isArray().
- Write a JavaScript function that checks if an object is iterable and has a length property indicative of an array.
- Write a JavaScript program that distinguishes arrays from objects by testing the constructor.
- Write a JavaScript function that uses Object.prototype.toString() to confirm if a value is an Array.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will check whether the given argument is a native boolean element.
Next: Write a JavaScript program to check if a given string is an anagram of another string (case-insensitive, ignores spaces, punctuation and special characters).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.