JavaScript: Determine whether the current runtime environment is a browser
JavaScript fundamental (ES6 Syntax): Exercise-203 with Solution
Check Browser Environment
Write a JavaScript program to determine if the current runtime environment is a browser. This is so that front-end modules can run on the server (Node) without errors.
- Use Array.prototype.includes() on the typeof values of both window and document (globals usually only available in a browser environment unless they were explicitly defined), which will return true if one of them is undefined.
- typeof allows globals to be checked for existence without throwing a ReferenceError.
- If both of them are not undefined, then the current environment is assumed to be a browser.
Sample Solution:
JavaScript Code:
// Define a function 'isBrowser' that checks if the code is running in a browser environment
const isBrowser = () => ![typeof window, typeof document].includes('undefined');
// Test cases to check if the code is running in a browser environment
console.log(isBrowser());
console.log(isBrowser());
Output:
true true
Flowchart:
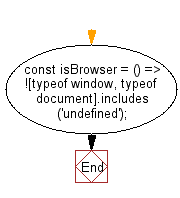
Live Demo:
See the Pen javascript-basic-exercise-203-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if the global window object is defined, indicating a browser environment.
- Write a JavaScript function that checks for the existence of document and navigator objects to confirm a browser.
- Write a JavaScript program that distinguishes between Node.js and browser environments using typeof window.
- Write a JavaScript function that safely accesses browser-specific objects and returns a boolean flag.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will return true whether the browser tab of the page is focused, false otherwise.
Next: Write a JavaScript program that will check whether the given argument is a native boolean element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.