JavaScript: Check whether the first numeric argument is divisible by the second one
JavaScript fundamental (ES6 Syntax): Exercise-201 with Solution
Check Divisibility
Write a JavaScript program to check if the first numerical argument is divisible by the second one.
- Use the modulo operator (%) to check if the remainder is equal to 0.
Sample Solution:
JavaScript Code:
// Define a function 'isDivisible' that checks if 'dividend' is divisible by 'divisor'
const isDivisible = (dividend, divisor) => dividend % divisor === 0;
// Test cases to check if 'dividend' is divisible by 'divisor'
console.log(isDivisible(6, 3)); // true (6 is divisible by 3)
console.log(isDivisible(5, 3)); // false (5 is not divisible by 3)
console.log(isDivisible(100, 10)); // true (100 is divisible by 10)
console.log(isDivisible(0, 3)); // true (0 is divisible by any non-zero number)
Output:
true false true true
Visual Presentation:
Flowchart:
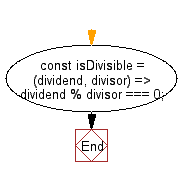
Live Demo:
See the Pen javascript-basic-exercise-201-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that checks if the first numerical argument is divisible by the second using the modulus operator.
- Write a JavaScript function that returns a boolean indicating whether one number divides another evenly.
- Write a JavaScript program that validates two numbers and logs if the first is completely divisible by the second.
- Write a JavaScript function that handles edge cases like division by zero when checking divisibility.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will return true if a value is an empty object, collection, map or set, has no enumerable properties or is any type that is not considered a collection.
Next: Write a JavaScript program to check if a given number is even or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.