JavaScript: Return true if a value is an empty object, collection, map or set, has no enumerable properties or is any type that is not considered a collection
JavaScript fundamental (ES6 Syntax): Exercise-200 with Solution
Check If Empty Object or Collection
Write a JavaScript program that returns true if a value is an empty object, collection, map or set. It has no enumerable properties or is of any type not considered a collection.
- Check if the provided value is null or if its length is equal to 0.
Sample Solution:
JavaScript Code:
// Define a function 'isEmpty' that checks if the given value 'val' is empty
const isEmpty = val =>
// Check if 'val' is null, undefined, an empty array, an empty object,
// an empty Map, an empty Set, or an empty string
val == null || !(Object.keys(val) || val).length;
// Test cases to check if the values are empty
console.log(isEmpty(new Map())); // true (the Map is empty)
console.log(isEmpty(new Set())); // true (the Set is empty)
console.log(isEmpty([])); // true (the array is empty)
console.log(isEmpty({})); // true (the object is empty)
console.log(isEmpty('')); // true (the string is empty)
console.log(isEmpty([1, 2])); // false (the array is not empty)
console.log(isEmpty({ a: 1, b: 2 })); // false (the object is not empty)
console.log(isEmpty('text')); // false (the string is not empty)
console.log(isEmpty(123)); // true (numbers are considered empty)
console.log(isEmpty(true)); // true (booleans are considered empty)
Output:
true true true true true false false false true true
Flowchart:
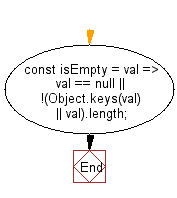
Live Demo:
See the Pen javascript-basic-exercise-200-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if an object has no enumerable properties.
- Write a JavaScript function that checks if an array, map, or set is empty by examining its length or size.
- Write a JavaScript program that returns true for empty objects, arrays, and collections, and false otherwise.
- Write a JavaScript function that determines if a value is an empty collection by iterating over its keys.
Go to:
PREV : Check If Even Number.
NEXT : Check Divisibility.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.