JavaScript: Copy a string to the clipboard
JavaScript fundamental (ES6 Syntax): Exercise-2 with Solution
Copy String to Clipboard
Write a JavaScript program to copy a string to the clipboard.
- Create a new <textarea> element, fill it with the supplied data and add it to the HTML document.
- Use Selection.getRangeAt()to store the selected range (if any).
- Use Document.execCommand('copy') to copy to the clipboard.
- Remove the <textarea> element from the HTML document.
- Finally, use Selection().addRange() to recover the original selected range (if any).
NOTICE: The same functionality can be easily implemented by using the new asynchronous Clipboard API, which is still experimental but should be used in the future instead of this snippet.
Sample Solution:
JavaScript Code:
//#Source https://bit.ly/2neWfJ2
const copy_to_Clipboard = str => {
const el = document.createElement('textarea');
el.value = str;
el.setAttribute('readonly', '');
el.style.position = 'absolute';
el.style.left = '-9999px';
document.body.appendChild(el);
const selected =
document.getSelection().rangeCount > 0 ? document.getSelection().getRangeAt(0) : false;
el.select();
document.execCommand('copy');
document.body.removeChild(el);
if (selected) {
document.getSelection().removeAllRanges();
document.getSelection().addRange(selected);
}
};
Flowchart:
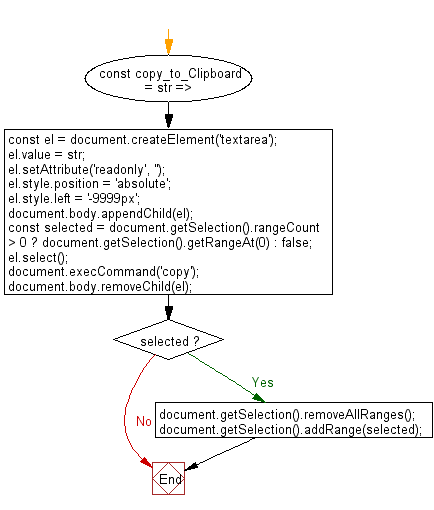
Live Demo:
See the Pen javascript-basic-exercise-1-2 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to compare two objects to determine if the first one contains equivalent property values to the second one.
Next: Write a JavaScript program to converts a comma-separated values (CSV) string to a 2D array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics