JavaScript: Check whether the provided value is an object created by the Object constructor
JavaScript fundamental (ES6 Syntax): Exercise-192 with Solution
Check If Object by Constructor
Write a JavaScript program to check whether the provided value is an object created by the Object constructor.
- Check if the provided value is truthy.
- Use typeof to check if it is an object and Object.prototype.constructor to make sure the constructor is equal to Object.
Sample Solution:
JavaScript Code:
// Define a function 'isPlainObject' that checks if the given value 'val' is a plain object
const isPlainObject = val =>
// Check if 'val' is not falsy and is an object, and its constructor is Object
!!val && typeof val === 'object' && val.constructor === Object;
// Test cases to check if the value is a plain object
console.log(isPlainObject({ a: 1 })); // true (the value is a plain object)
console.log(isPlainObject(new Map())); // false (the value is not a plain object)
Output:
true false
Flowchart:
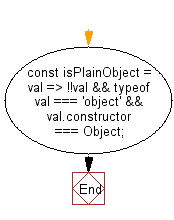
Live Demo:
See the Pen javascript-basic-exercise-192-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that verifies if a value is an object created by the Object constructor.
- Write a JavaScript function that tests whether an input is a plain object and not an instance of a custom class.
- Write a JavaScript program that uses Object.getPrototypeOf() to confirm that a value’s constructor is Object.
- Write a JavaScript function that distinguishes between plain objects and other object types using constructor checks.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to check whether the provided integer is a prime number or not.
Next: Write a JavaScript program to check if a value is object-like. Check whether the provided value is not null and its typeof is equal to 'object'.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.