JavaScript: Return true if an object looks like a Promise, false otherwise
JavaScript fundamental (ES6 Syntax): Exercise-189 with Solution
Check If Looks Like Promise
Write a JavaScript program that returns true if an object looks like a Promise, false otherwise.
- Check if the object is not null, its typeof matches either object or function and if it has a .then property, which is also a function.
Sample Solution:
JavaScript Code:
// Define a function 'isPromiseLike' that checks if the given object 'obj' is similar to a Promise
const isPromiseLike = obj =>
// Check if the object is not null and has a 'then' method, typically present in Promise objects
obj !== null &&
(typeof obj === 'object' || typeof obj === 'function') &&
typeof obj.then === 'function';
// Test cases to check if the objects are promise-like
console.log(isPromiseLike({
then: function() {
return '';
}
})); // true (has 'then' method)
console.log(isPromiseLike(null)); // false (null)
console.log(isPromiseLike({})); // false (object without 'then' method)
Output:
true false false
Flowchart:
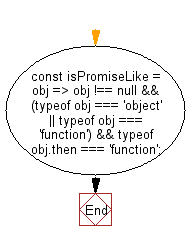
Live Demo:
See the Pen javascript-basic-exercise-189-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns true if an object has a then method and appears to be a Promise.
- Write a JavaScript function that checks if an input is “thenable” by testing for the presence of a callable then property.
- Write a JavaScript program that inspects an object’s structure to determine if it follows the Promise interface.
- Write a JavaScript function that uses duck typing to verify if an object behaves like a Promise.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program that will return 1 if the array is sorted in ascending order, -1 if it is sorted in descending order or 0 if it is not sorted.
Next: Write a JavaScript program to get a boolean determining if the passed value is primitive or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.