JavaScript: Check if a given string is upper case or not
JavaScript fundamental (ES6 Syntax): Exercise-184 with Solution
Check String Uppercase
Write a JavaScript program to check if a given string is upper case or not.
- Convert the given string to upper case, using String.prototype.toUpperCase() and compare it to the original.
Sample Solution:
JavaScript Code:
// Define a function 'isUpperCase' that checks if all characters in a string 'str' are uppercase
const isUpperCase = str => str === str.toUpperCase();
// Test cases to check if the provided strings contain only uppercase characters
console.log(isUpperCase('ABC')); // true (all characters are uppercase)
console.log(isUpperCase('A3@$')); // true (all characters are uppercase)
console.log(isUpperCase('aB4')); // false (contains lowercase characters)
Output:
true true false
Visual Presentation:
Flowchart:
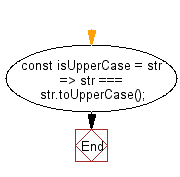
Live Demo:
See the Pen javascript-basic-exercise-184-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that determines if an entire string is in uppercase by comparing it to its uppercase version.
- Write a JavaScript function that tests a string and returns true if all alphabetic characters are uppercase.
- Write a JavaScript program that ignores non-letter characters and checks if the remaining letters are all in uppercase.
- Write a JavaScript function that converts a string to uppercase and then compares it with the original to determine its case.
Go to:
PREV : Check Valid JSON.
NEXT : Check If Undefined.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.