JavaScript: Check whether the provided argument is a valid JSON
JavaScript fundamental (ES6 Syntax): Exercise-183 with Solution
Check Valid JSON
Write a JavaScript program to check if the provided argument is valid JSON.
- Use JSON.parse() and a try... catch block to check if the provided string is a valid JSON.
Sample Solution:
JavaScript Code:
// Define a function 'isValidJSON' that checks if a given string 'obj' is a valid JSON
const isValidJSON = obj => {
// Try to parse the string as JSON
try {
JSON.parse(obj);
// If parsing succeeds, return true
return true;
} catch (e) {
// If parsing fails (due to invalid JSON), return false
return false;
}
};
// Test cases to check if the provided strings are valid JSON or not
console.log(isValidJSON('{"name":"Adam","age":20}')); // true (valid JSON)
console.log(isValidJSON('{"name":"Adam",age:"20"}')); // false (invalid JSON)
console.log(isValidJSON(null)); // false (not a string)
Output:
true false true
Flowchart:
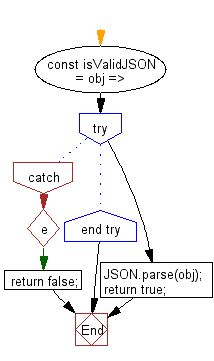
Live Demo:
See the Pen javascript-basic-exercise-183-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that tests whether a given string is valid JSON using try/catch and JSON.parse().
- Write a JavaScript function that returns a boolean indicating if an input string can be successfully parsed as JSON.
- Write a JavaScript program that validates a JSON string and logs detailed error messages if parsing fails.
- Write a JavaScript function that checks for proper JSON structure and returns either the parsed object or an error flag.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to join all elements of an array into a string and returns this string.
Next: Write a JavaScript program to check if a given string is upper case or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.