JavaScript: Join all elements of an array into a string and returns this string
JavaScript fundamental (ES6 Syntax): Exercise-182 with Solution
Write a JavaScript program to join all elements of an array into a string and return this string. Use a separator and an end separator.
- Use Array.prototype.reduce() to combine elements into a string.
- Omit the second argument, separator, to use a default separator of ','.
- Omit the third argument, end, to use the same value as separator by default.
Sample Solution:
JavaScript Code:
// Define a function 'join' that concatenates elements of an array 'arr' into a single string
// with specified 'separator' between elements and 'end' at the end of the string
const join = (arr, separator = ',', end = separator) =>
// Reduce the array into a single string
arr.reduce(
(acc, val, i) =>
// If it's the second last element, concatenate 'end' instead of 'separator'
i === arr.length - 2
? acc + val + end
// If it's the last element, concatenate only the value without 'separator' or 'end'
: i === arr.length - 1
? acc + val
// Concatenate the value with 'separator'
: acc + val + separator,
'' // Start with an empty string
);
// Print the joined string with specified separator ',' and end '&'
console.log(join(['pen', 'pineapple', 'apple', 'pen'], ',', '&'));
// Print the joined string with specified separator ','
console.log(join(['pen', 'pineapple', 'apple', 'pen'], ','));
// Print the joined string with default separator ',' and default end ','
console.log(join(['pen', 'pineapple', 'apple', 'pen']));
Output:
pen,pineapple,apple&pen pen,pineapple,apple,pen pen,pineapple,apple,pen
Visual Presentation:
Flowchart:
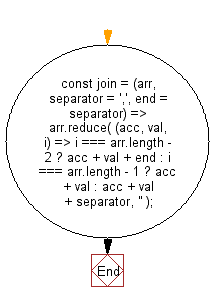
Live Demo:
See the Pen javascript-basic-exercise-182-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the last element from an given array.
Next: Write a JavaScript program to check if the provided argument is a valid JSON.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics