JavaScript: Get the last element from a given array
JavaScript fundamental (ES6 Syntax): Exercise-181 with Solution
Get Last Array Element
Write a JavaScript program to get the last element from a given array.
- Check if arr is truthy and has a length property.
- Use Array.prototype.length - 1 to compute the index of the last element of the given array and return it, otherwise return undefined.
Sample Solution:
JavaScript Code:
// Define a function 'last' that takes an array 'arr' as input
const last = arr =>
// Return the last element of the input array
arr[arr.length - 1];
// Print the last element of the array [1, 2, 3]
console.log(last([1, 2, 3]));
// Print the last element of the array [1, 2, 3, 4, 5, 7]
console.log(last([1, 2, 3, 4, 5, 7]));
Output:
3 7
Visual Presentation:
Flowchart:
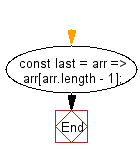
Live Demo:
See the Pen javascript-basic-exercise-181-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that returns the last element of an array using array indexing.
- Write a JavaScript function that retrieves the final element of an array and handles empty arrays gracefully.
- Write a JavaScript program that uses the slice() method to extract the last element from an array.
- Write a JavaScript function that returns the last item of an array and verifies its existence before accessing it.
Go to:
PREV : Object with Lowercase Keys.
NEXT : Join Array with End Separator.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.