JavaScript: Create a new object from the specified object, where all the keys are in lowercase
JavaScript fundamental (ES6 Syntax): Exercise-180 with Solution
Object with Lowercase Keys
Write a JavaScript program to create an object from the specified object, where all keys are in lowercase.
- Use Object.keys() and Array.prototype.reduce() to create a new object from the specified object.
- Convert each key in the original object to lowercase, using String.prototype.toLowerCase().
Sample Solution:
JavaScript Code:
// Define a function 'lowercaseKeys' that takes an object 'obj' as input
const lowercaseKeys = obj =>
// Use 'reduce' to iterate over the keys of the object and create a new object with lowercase keys
Object.keys(obj).reduce((acc, key) => {
// Set the key in the new object to lowercase and assign the corresponding value from the original object
acc[key.toLowerCase()] = obj[key];
// Return the updated accumulator object
return acc;
}, {});
// Define an object 'myObj' with keys in mixed case
const myObj = { Name: 'Adam', sUrnAME: 'Smith' };
// Call 'lowercaseKeys' function with 'myObj' and assign the result to 'myObjLower'
const myObjLower = lowercaseKeys(myObj);
// Print the resulting object with lowercase keys
console.log(myObjLower);
Output:
{"name":"Adam","surname":"Smith"}
Visual Presentation:
Flowchart:
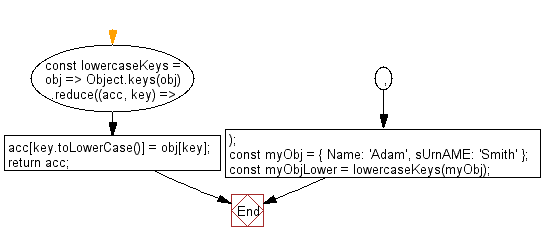
Live Demo:
See the Pen javascript-basic-exercise-180-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that converts all keys of an object to lowercase and returns a new object with unchanged values.
- Write a JavaScript function that iterates over an object and constructs a new object with each key transformed to lowercase.
- Write a JavaScript program that deep clones an object while converting all nested keys to lowercase.
- Write a JavaScript function that checks an object’s keys and outputs a version with all keys normalized to lowercase.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to compare two objects to determine if the first one contains equivalent property values to the second one, based on a provided function.
Next: Write a JavaScript program to get the last element from an given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.