JavaScript: Compare two objects to determine if the first one contains equivalent property values to the second one, based on a provided function
JavaScript fundamental (ES6 Syntax): Exercise-179 with Solution
Object Comparison by Function
Write a JavaScript program to compare two objects to determine if the first contains equivalent property values to the second one. This is based on a provided function.
- Use Object.keys() to get all the keys of the second object.
- Use Array.prototype.every(), Object.prototype.hasOwnProperty() and the provided function to determine if all keys exist in the first object and have equivalent values.
- If no function is provided, the values will be compared using the equality operator.
Sample Solution:
JavaScript Code:
// Define a function 'matchesWith' that takes three arguments: 'obj', 'source', and 'fn'
const matchesWith = (obj, source, fn) =>
// Check if every key in 'source' satisfies a condition
Object.keys(source).every(
// Callback function for 'every' with parameters 'key' representing the current key
key =>
// Check if 'obj' has the key 'key' and whether 'fn' exists
obj.hasOwnProperty(key) && fn
// If both conditions are true, call the function 'fn' with parameters
? fn(obj[key], source[key], key, obj, source)
// If 'fn' doesn't exist, compare the values of 'obj[key]' and 'source[key]'
: obj[key] == source[key] // If they are equal, return true; otherwise, return false
);
// Define a function 'isGreeting' that checks if a string is a greeting ('hi' or 'hello')
const isGreeting = val => /^h(?:i|ello)$/.test(val);
// Call 'matchesWith' with two objects and a custom comparison function
console.log(matchesWith(
{ greeting: 'hello' }, // Object 'obj' with property 'greeting' set to 'hello'
{ greeting: 'hi' }, // Object 'source' with property 'greeting' set to 'hi'
// Custom comparison function to check if both values are greetings using 'isGreeting' function
(oV, sV) => isGreeting(oV) && isGreeting(sV)
));
Output:
true
Flowchart:
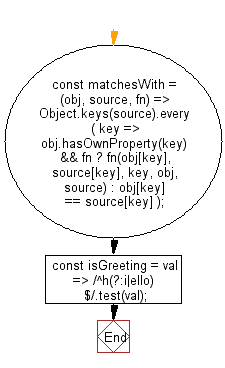
Live Demo:
See the Pen javascript-basic-exercise-179-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that compares two objects using a custom comparator function for their property values.
- Write a JavaScript function that accepts a comparison callback to determine if one object’s values are equivalent to another’s.
- Write a JavaScript program that recursively compares two objects for equality based on a user-supplied testing function.
- Write a JavaScript function that verifies object equivalence by applying a comparator to each corresponding property pair.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create a new object from the combination of two or more objects.
Next: Write a JavaScript program to create a new object from the specified object, where all the keys are in lowercase.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics