JavaScript: Create a new object from the combination of two or more objects
JavaScript fundamental (ES6 Syntax): Exercise-178 with Solution
Merge Objects into New Object
Write a JavaScript program to create a new object from the combination of two or more objects.
- Use Array.prototype.reduce() combined with Object.keys() to iterate over all objects and keys.
- Use Object.prototype.hasOwnProperty() and Array.prototype.concat() to append values for keys existing in multiple objects.
Sample Solution:
JavaScript Code:
// Define a function 'merge' that takes any number of objects as arguments using the rest parameter syntax '...objs'
const merge = (...objs) =>
// Use the spread operator to create a shallow copy of the 'objs' array, then use 'reduce' to merge the objects
[...objs].reduce(
// Callback function for 'reduce' with accumulator 'acc' and current object 'obj'
(acc, obj) =>
// Reduce the keys of the current object 'obj' into the accumulator 'acc' using another 'reduce' function
Object.keys(obj).reduce(
// Callback function for inner 'reduce' with accumulator 'a' and current key 'k'
(a, k) => {
// Check if the accumulator 'acc' already has the key 'k'
acc[k] = acc.hasOwnProperty(k) ? // If yes, concatenate the values of 'acc[k]' and 'obj[k]' arrays or add the value 'obj[k]' to 'acc[k]'
[].concat(acc[k]).concat(obj[k]) : obj[k];
return acc; // Return the updated accumulator 'acc'
},
{} // Initial value of the inner 'reduce' accumulator is an empty object
),
{} // Initial value of the outer 'reduce' accumulator is an empty object
);
// Define two objects 'object' and 'other' with properties 'a', 'b', and 'c'
const object = {
a: [{ x: 2 }, { y: 4 }],
b: 1
};
const other = {
a: { z: 3 },
b: [2, 3],
c: 'foo'
};
// Merge the two objects using the 'merge' function and log the result
console.log(merge(object, other));
Output:
{"a":[{"x":2},{"y":4},{"z":3}],"b":[1,2,3],"c":"foo"}
Flowchart:
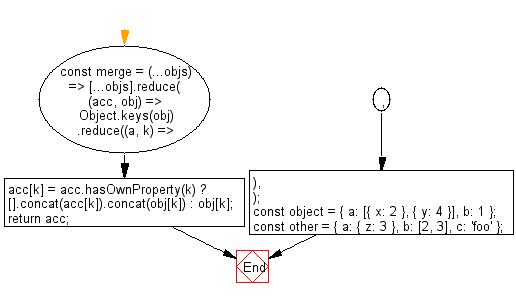
Live Demo:
See the Pen javascript-basic-exercise-178-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the minimum value of an array, after mapping each element to a value using the provided function.
Next: Write a JavaScript program to compare two objects to determine if the first one contains equivalent property values to the second one, based on a provided function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics