JavaScript: Get the minimum value of an array, after mapping each element to a value using the provided function
JavaScript fundamental (ES6 Syntax): Exercise-177 with Solution
Minimum Value After Mapping
Write a JavaScript program to get the minimum value of an array, after mapping each element to a value using the provided function.
- Use Array.prototype.map() to map each element to the value returned by fn.
- Use Math.min() to get the minimum value.
Sample Solution:
JavaScript Code:
// Define a function 'minBy' that takes an array 'arr' and a function 'fn'
const minBy = (arr, fn) =>
// Use the spread operator to pass each element of the mapped array as arguments to Math.min
Math.min(
// Map each element of the input array 'arr' to the value returned by 'fn' or the value of 'n' property if 'fn' is a string
...arr.map(typeof fn === 'function' ? fn : val => val[fn])
);
// Log the minimum value of the 'n' property of objects in the array [{ n: 4 }, { n: 2 }, { n: 8 }, { n: 6 }]
console.log(minBy([{ n: 4 }, { n: 2 }, { n: 8 }, { n: 6 }], o => o.n));
// Log the minimum value of the 'n' property of objects in the array [{ n: 4 }, { n: 2 }, { n: 8 }, { n: 6 }]
console.log(minBy([{ n: 4 }, { n: 2 }, { n: 8 }, { n: 6 }], 'n'));
Output:
2 2
Visual Presentation:
Flowchart:
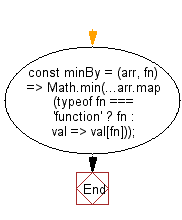
Live Demo:
See the Pen javascript-basic-exercise-177-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that maps each element of an array through a provided function and returns the minimum resulting value.
- Write a JavaScript function that transforms an array and then applies Math.min to the transformed values.
- Write a JavaScript program that iterates over an array, applies a callback to each element, and tracks the smallest output.
- Write a JavaScript function that uses reduce() to compute the minimum value after applying a mapping function.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the n minimum elements from the provided array.
Next: Write a JavaScript program to create a new object from the combination of two or more objects.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.