JavaScript: Get the n minimum elements from the provided array
JavaScript fundamental (ES6 Syntax): Exercise-176 with Solution
n Minimum Elements in Array
Write a JavaScript program to get the n minimum elements from the provided array.
If n is greater than or equal to the provided array's length, return the original array (sorted in ascending order).
- Use Array.prototype.sort() combined with the spread operator (...) to create a shallow clone of the array and sort it in ascending order.
- Use Array.prototype.slice() to get the specified number of elements.
- Omit the second argument, n, to get a one-element array.
- If n is greater than or equal to the provided array's length, then return the original array (sorted in ascending order).
Sample Solution:
JavaScript Code:
// Define a function 'minN' that takes an array 'arr' and an optional parameter 'n' with a default value of 1
const minN = (arr, n = 1) =>
// Create a shallow copy of the array 'arr', sort it in ascending order, and then extract the first 'n' elements
[...arr].sort((a, b) => a - b).slice(0, n);
// Log the smallest element from the array [1, 2, 3]
console.log(minN([1, 2, 3]));
// Log the smallest '2' elements from the array [1, 2, 3]
console.log(minN([1, 2, 3], 2));
Output:
[1] [1,2]
Visual Presentation:
Flowchart:
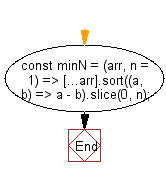
Live Demo:
See the Pen javascript-basic-exercise-176-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the index of the function in an array of functions which executed the fastest.
Next: Write a JavaScript program to get the minimum value of an array, after mapping each element to a value using the provided function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics