JavaScript: Get the index of the function in an array of functions which executed the fastest
JavaScript fundamental (ES6 Syntax): Exercise-175 with Solution
Index of Fastest Function
Write a JavaScript program to get the index of the function in an array of functions which executed the fastest.
- Use Array.prototype.map() to generate an array where each value is the total time taken to execute the function after iterations times.
- Use the difference in performance.now() values before and after to get the total time in milliseconds to a high degree of accuracy.
- Use Math.min() to find the minimum execution time, and return the index of that shortest time which corresponds to the index of the most performant function.
- Omit the second argument, iterations, to use a default of 10000 iterations.
- The more iterations, the more reliable the result but the longer it will take.
Sample Solution:
JavaScript Code:
// Define a function 'mostPerformant' that takes an array of functions 'fns' and an optional parameter 'iterations' with a default value of 10000
const mostPerformant = (fns, iterations = 10000) => {
// Map each function in 'fns' to its execution time in milliseconds
const times = fns.map(fn => {
// Record the current timestamp in milliseconds before executing the function
const before = performance.now();
// Execute the function 'iterations' number of times
for (let i = 0; i < iterations; i++) fn();
// Calculate the elapsed time by subtracting the start time from the current time
return performance.now() - before;
});
// Find the index of the minimum time in the 'times' array and return it
return times.indexOf(Math.min(...times));
};
// Log the index of the most performant function among the provided functions
console.log(mostPerformant([
// First function: Loops through the entire array before returning `false`
() => {
[1, 2, 3, 4, 5, 6, 7, 8, 9, '10'].every(el => typeof el === 'number');
},
// Second function: Only needs to reach index `1` before returning false
() => {
[1, '2', 3, 4, 5, 6, 7, 8, 9, 10].every(el => typeof el === 'number');
}
])); // 1
Output:
1
Flowchart:
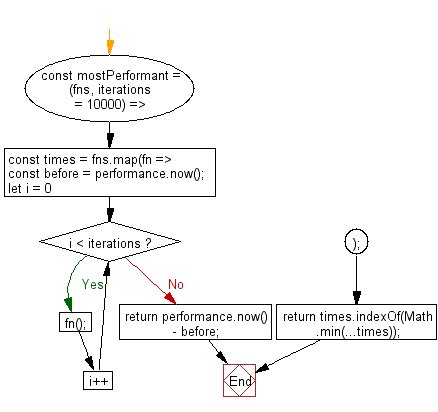
Live Demo:
See the Pen javascript-basic-exercise-174-1 by w3resource (@w3resource) on CodePen.
For more Practice: Solve these Related Problems:
- Write a JavaScript program that times an array of functions and returns the index of the function with the fastest execution time.
- Write a JavaScript function that measures execution time of each function in a list and compares their durations.
- Write a JavaScript program that runs multiple functions with the same input and determines which completes first.
- Write a JavaScript function that uses performance.now() to record execution times and identifies the quickest function.
Go to:
PREV : Convert NodeList to Array.
NEXT : n Minimum Elements in Array.
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.