JavaScript: Get the nth element of a given array
JavaScript fundamental (ES6 Syntax): Exercise-173 with Solution
Get nth Array Element
Write a JavaScript program to get the nth element of a given array of elements.
- Use Array.prototype.slice() to get an array containing the nth element at the first place.
- If the index is out of bounds, return undefined.
- Omit the second argument, n, to get the first element of the array.
Sample Solution:
JavaScript Code:
// Define a function 'nthElement' that takes an array 'arr' and an optional parameter 'n' (defaulted to 0)
const nthElement = (arr, n = 0) =>
// If 'n' is positive, return a new array containing the element at index 'n'
// If 'n' is negative, return a new array containing the element at index 'n' from the end of the array
// If 'n' is zero or not provided, return the first element of the array
(n > 0 ? arr.slice(n, n + 1) : arr.slice(n))[0];
// Log the element at index 1 of the array ['a', 'b', 'c']
console.log(nthElement(['a', 'b', 'c'], 1)); // 'b'
// Log the element at index -3 of the array ['a', 'b', 'b']
console.log(nthElement(['a', 'b', 'b'], -3)); // 'a'
Output:
b a
Visual Presentation:
Flowchart:
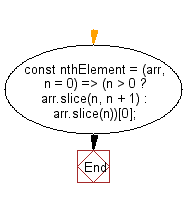
Live Demo:
See the Pen javascript-basic-exercise-173-1 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to create a function that invokes the provided function with its arguments transformed.
Next: Write a JavaScript program to convert a NodeList to an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics